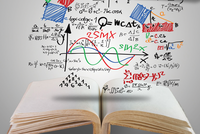
Lead Image © Buchachon Petthanya, 123RF.com
Symbolic mathematics with Python's SymPy library
Complex Math
Article from ADMIN 48/2018
The Python SymPy library for symbolic mathematics allows you to create complex mathematical expressions.
SymPy [1] is a computer algebra system written in the Python programming language. Among its many features are algorithms for computing derivatives, integrals, and limits; functions for manipulating and simplifying expressions; functions for symbolically solving equations and ordinary and partial differential equations; two- and three-dimensional (2D and 3D) plotting; and much more (e.g., see the SymPy features list [2] and Table 1 in a PeerJ Computer Science paper [3]).
...Use Express-Checkout link below to read the full article (PDF).
Buy this article as PDF
Express-Checkout as PDF
Price $2.95
(incl. VAT)
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
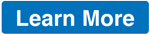