« Previous 1 2 3 4 Next »
Requirements for centralized password management
Well Secured?
Typing in login names and passwords has, for many years, been the most common form of authentication in IT environments with normal protection requirements. Alternatives such as tokens, smart cards, electronic cards, and various types of biometrics have not changed this. There is virtually no alternative to passwords: Low implementation costs, sufficiently high user acceptance, and the relative rarity of significant security incidents suggest that passwords are unlikely to become extinct in the near future.
Emergency password management covers situations in which third parties not directly involved in service operations need access to systems under exceptional circumstances to prevent greater damage. For example, the objective could be for a 24/7 security team to access compromised servers, even if the system administrator is not available.
In Case of Emergency
The classic solution to this problem is a list of passwords in a sealed envelope that is deposited in a vault and handed over when an emergency occurs. Conventional solutions like this, however, do not scale adequately: Now larger organizations and data centers need to deposit not just a handful but dozens or hundreds of passwords, and regular password changes are required not only for password policies based on ISO/IEC 27001 but also, for example, in case of staff changes.
Maintaining a stored list of passwords thus evolves from a subjectively annoying chore to an objective time waster. Migrating emergency password management to a centralized, server-based software solution that can be used from any workstation offers many benefits but also incurs many security risks and needs to be well considered because of its importance.
This article examines the opportunities and risks and derives specific selection criteria for centralized password management products. The practical implementation is discussed using the Leibniz
...Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
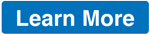