« Previous 1 2
Programming with Node.js and JavaScript
Turbulent World
In web development, trends quickly come and go. For a time, Ruby on Rails was all the rage; now the hype has shifted away from the framework and is focused on JavaScript, the only programming language to run on the client and on the server, if you subscribe to the credo of JavaScript's followers.
Server-side JavaScript frameworks have been around for a while, such as Helma [1], on which the website of the Austrian Broadcasting Corporation (ORF) is based. Helma is slowly being mothballed, but a successor named Ringo is already in the starting blocks. Projects like these, however, have only ever been niche products compared with Rails and PHP frameworks.
The situation is different with the Node.js platform (or Node for short) [2], which has been extremely popular for several years and has attracted a large community of developers. Node was developed by Ryan Dahl, who coupled Google's V8 JavaScript compiler with some non-blocking C libraries whose functions are available in JavaScript. This approach allows event-based processing of work requests and, thus, more concurrent requests than the Apache server can handle – although Apache also has an event-based processing module [3] in its more recent incarnations.
Spoiled for Choice
One of the strengths of Node.js, and a result of its massive popularity, is the huge number of modules that extend its functionality. The hype now goes so far that Node is no longer limited to web applications but is even used for classic system tools. Thus, the administration tools in SmartOS [4] are based on Node – which is perhaps less surprising if you know that Joyent, the company behind SmartOS, is involved in the Node project and sees
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
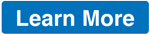