« Previous 1 2 3 4 Next »
Developing RESTful APIs
Late Bloomer
Almost 15 years before Tim Berners-Lee and Robert Cailliau started up the first World Wide Web (WWW) server, James E. White published the idea of the Remote Procedure Call (RPC) with Request for Comments (RFC) 707 [1] in 1976. The idea was for an RPC to allow a computer to run a function on a remote computer. Machine-to-machine communication (M2M) thus became real.
In contrast, while developing the HTTP protocol and the hypertext markup language (HTML), Berners-Lee was thinking of human users who navigate globally distributed documents – that is, the man-machine interface.
The main difference between the two architectures is how the client approaches the resources on the server. RPC requires the client to possess very specific knowledge of how to access remote resources on the server. Here, the developer needs to study the protocol specification in detail to understand the meaning of the data elements for input and output.
On the web, however, a visitor is unlikely to read the instructions for a web page before using the service, thanks to the text-based HTML format. Users intuitively conclude how to use a web page from what they see on the page itself. Machines do things differently. In M2M communication, the developer needs to define how the machine interprets the data in the form of specifications.
Back in 2000, Roy T. Fielding, in his highly readable thesis [2], called for a drive to establish the four principles of high scalability, ease of usability, extensibility, and self-descriptive hypermedia as the basis for an efficient web architecture and to implement it consistently in the form of Representational State Transfer (REST).
Machine Talk
The inventors of the WWW quickly realized that humans were not the only ones who could take advantage of the
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
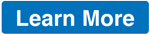