Modularize websites with Web Components
Web Perfect
Microservices are being used more and more as architecture patterns for client-server applications. Developers break down monolithic back ends into smaller services that can be developed independently of each other and, if necessary, re-implemented with little effort.
Web developers naturally ask themselves how they can best integrate the multitude of small services into their websites. One answer is with Web Components [1], self-adjustable and reusable HTML tags that are standardized, work across browsers, and can be used with all JavaScript libraries and frameworks that also work with HTML (see Table 1).
Table 1
Native Use of Web Components
Browser | Version | Example Works? |
---|---|---|
Chrome | 69 | Yes |
Firefox | 63 | Yes |
Safari | 12 | Yes (untested) |
MS Edge | 42 | No |
For example, developers can use Web Components to integrate microservices into
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
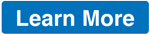