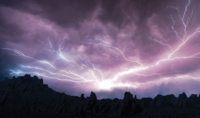
Photo by Micah Tindell on Unsplash
Have a Bash with the Zing network utility
Zinger
Zing is a zero-packet network utility similar to Ping that I first introduced in December 2022 [1]. Zing has the distinct advantage that hosts without Ping capability can be zinged without sending network packets. Thus, zinging a remote host with multiple zing
requests is not a "Ping flood" [2] attack, making Zing a network-safe utility that does not add network traffic.
Zing is implemented in a variety of programming languages, is open source, and is available for download [3]. Yet the idea occurred to me to implement zing
as a Bash shell script, which has the advantage of avoiding recompilation and of customization or tailoring for specific idiosyncrasies of a system platform or application.
Netcat
The netcat utility (nc
) is a key command-line application and "Swiss army knife" of network utilities [4]. Netcat is described as a simple Unix utility that reads and writes data across network connections by the TCP or UDP protocol [5]. Originally developed for Unix, netcat has since been ported to many other computer platforms. Thus, netcat has the advantage of being somewhat ubiquitous across different operating systems.
The decision to implement zing
with netcat in a shell script left one major design consideration decision. Put simply: Which shell to use? I develop on macOS, so Zsh seemed a likely obvious choice. However, I also work with Linux, so other shells were possible.
Ultimately I opted to go with Bash because it is ubiquitous, and I have done much more scripting for work and tinkering with the Bash shell. Moreover, Bash scripting
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
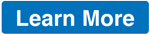