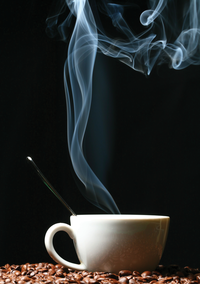
Lead Image © Ivan Mikhaylov, 123RF.com
Blending Java with other programming languages
It's in the Blend
Neither project managers nor programmers mix languages purely for pleasure. The former are worried about the complexity and the increased demands on their team, while the latter prefer to use things that they feel most comfortable with – and that is precisely one programming language for most of them. Having said this, the second programming language often does more good than harm in a software project:
- Bazaar method: The more expensive the software, the more benefits you have from leveraging tried and trusted routines – even if the recycled code is written in a different language.
- Performance: If you need low-level access to hardware and operating system resources, low-level programming languages offer superior performance.
- Scripting: Scripts and scripting languages give power users powerful tools. This is still the case with complex enterprise applications based on J2EE.
- Integration capability: Java applications can act as one component in the greater context and may need to adapt to match the look and feel of a desktop.
Java is a very powerful language, so it comes as no surprise that it also supports integration of software written in third-party languages for any use case. The following sections look into these techniques, along with their benefits and drawbacks.
Nothing Works Without C
One reason for Java's success is that it protects the developers from a few tricky things that were required in C and C++, in particular dynamic memory management. Despite this, the makers of Java saw from the outset that Java would have difficulty surviving in the real world without being able to access the versatile resources of existing C libraries. Thus, even the very first Java version offered the option of integrating C code in the form of the Java Native Interface (JNI).
Java access to C relies on two layers (
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
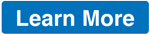