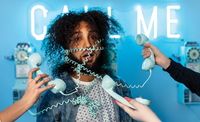
Photo by NoWah Bartscher on Unsplash
Gatling load-testing tool
Stressed
When questioned at work about how the test tool Gatling [1] compared with the somewhat old JMeter, I was at a loss for words. However, it did give me the opportunity to learn more about Gatling and eventually present another possible tool in the arsenal against weak servers and services.
In retrospect, I'm not sure how I missed Gatling, which is no recent upstart. Gatling's first stable release was in 2012, and only a few years later, its founder created Gatling Corp. to develop and maintain the software. Over the years, Gatling morphed into a product with both open source and Enterprise variants that can hold their own against other test tools.
Yet, Gatling has taken a significantly different path from some of the other load-testing products such as JMeter and LoadRunner. With Gatling, you don't have a custom IDE for development. Instead, you use the Gatling framework in your favorite editor to write your own test script in Scala.
Getting Started
All Gatling tests start pretty much the same, by subclassing from the Scala Simulation
class. The simulation is essentially a collection of individual requests that a user or program would normally make. Because your test is a program, you are given the flexibility of creating variables, constants, and methods, and because you are using a proper programming language, you can create your own library of support objects or methods.
Your performance tests can include one or more classes, but you can create and include a lot of regular Scala objects, as well. Scala also supports an object type that appears to be similar to a class, but it is more akin to a singleton than a normal Java or C++ class.
You don't have to be a Scala programmer to enjoy the freedom that Gatling provides. Most developers, despite having a favorite language or toolset, can easily learn
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
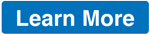