« Previous 1 2 3 Next »
Single sign-on like the big guys
Authenticate Anything
Once your set of internal applications grows greater than an order of 10s, you can end up in a scenario where credentials storage for each service gets out of control. Users might start complaining about how difficult it is to handle multiple passwords, and your day could turn into a password reset ticket nightmare. If you wonder whether a single sign-on (SSO) experience à la Google and Amazon is possible, even at a smaller scale, the answer is "Yes"! Keycloak can do exactly that.
A comprehensive administration introduction to Keycloak appeared previously in ADMIN [1], so in this article you will travel through the other end of the spectrum: How to enable your application with proper SSO, with or without writing code.
The Keycloak Project
Keycloak is a mature free and open source software (FOSS) project whose first production release goes back to the year 2014 [2]. It's largely funded and developed by Red Hat, and it is the software on which their SSO commercial offering is based. The tool's goal is to provide a modern and secure SSO experience for any application on the basis of either the OIDC or SAML framework (see the "OIDC vs. SAML" box).
OIDC vs. SAML
OpenID Connect (OIDC) is the only authentication framework used in this article, although Security Assertion Markup Language (SAML) is widely used and supported, especially in the Enterprise segment. The choice usually falls on OIDC because of its increasing popularity, lightness, and simplifications like data exchange by JSON instead of XML.
Until version 16, inclusive, Keycloak ran on top of the WildFly application server (formerly JBoss). Since version 17,
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
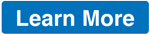