« Previous 1 2
Implement your own MIBs with Python
DIY MIBs
If you think of Wikipedia first when considering a freely expandable, hierarchical knowledge database, you are not totally wrong, but you have overlooked one classic example: The Simple Network Management Protocol (SNMP). This time-honored protocol can also conjure up a very wide range of very different information through a formalized protocol and Management Information Base (MIBs) modules.
MIBs are readily available from device and software manufacturers, but application developers looking to expose the internal metrics of their software can create them, as well. They can be equally useful for sys admins who want to monitor complex infrastructure setups such as DNSSEC. At first glance, implementing your own MIB might seem complex, but with the right tools, it's not that difficult. This article shows how to create a MIB for monitoring the system-on-a-chip (SoC) temperature of a Raspberry Pi with an easy-to-use Python module.
Management Information Base
SNMP can be compared in some ways to the Lightweight Directory Access Protocol (LDAP), another Internet classic. Both are network protocols that describe how to access information. In the case of LDAP, you consult an LDAP directory, which can take very different forms. In the case of SNMP, the Management Information Base that is an aggregation of implemented MIB modules can contain a wide range of information, from routing tables to the utilization level of filesystems, and from fan speeds to configuration states.
It's probably best to imagine the MIB as a collection of carefully defined objects and messages – in SNMP-speak this is Managed Objects and Notifications – that form a tree-like structure below a root node. Both are identified by their respective position in this tree through an object identifier (OID), which describes the path from the root node along the branches to the managed object or notification.
Each OID
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
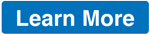