« Previous 1 2 3 4 Next »
New features in PHP 8
Conversion Work
One of the major innovations in PHP is intended to give the scripting language a boost, but it does require some prior knowledge. As of version 5.5, the code to be executed has been stored in a cache provided by the OPcache extension [1]. Thanks to this cache, the interpreter does not have to reread the scripts for every request.
PHP 8 sees OPcache expanded to include a just-in-time (JIT) compiler. This converts parts of the PHP code into native program code, which the processor then executes directly and far faster as a consequence. As a further speed tweak, the generated binary code is also cached. When the PHP code is restarted, PHP 8 simply accesses the precompiled binary code from the cache.
As the first benchmarks by PHP developer Brent Roose show [2], scripts with repetitive or extensive calculations obviously benefit from these changes. When handling individual short requests, the JIT compiler shows its advantages to a far lesser extent.
Abbreviation
The syntax, which has changed slightly in some places in PHP 8, saves developers some typing. For example, the scripting language adds a more powerful and more compact match
function to supplement switch
. In Listing 1, $flasher
is only On
if $lever
is set to Up
or Down
. If none of the comparison values before =>
are correct, the default
value applies.
Listing 1
match
$flasher = match ($lever) { 0 => "Warning_Flasher", 'Up', 'Down' => "On", default =>
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
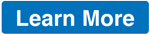