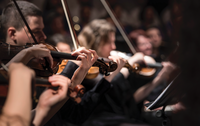
Photo by Larisa Birta on Unsplash
Convert Linux shell commands into PowerShell cmdlets
New Harmonies
PowerShell Crescendo [1] converts commands from other shell environments into PowerShell cmdlets and modules and runs them with all the benefits and convenience of the PowerShell environment. These commands can be piped or controlled with parameters. In this article, I show you how to set up and use a Crescendo environment in Linux.
Crescendo was available as a Technical Preview at the time of writing, so all of the approaches discussed here may change in future releases. However, because a Crescendo package and the wrappers it generates work without an Internet connection once downloaded, your environment can remain stable by not applying updates or not transpiling the wrappers again.
Crescendo Work Environment
Crescendo relies on a two-step process: Before using a command-line tool, you need to transpile a wrapper with the Crescendo environment and one or more JSON control files. If this is successful, the result will be an ordinary PowerShell module that you can use in pretty much any PowerShell version. This two-pronged approach is also reflected in the system requirements: To create a package based on Crescendo, you need PowerShell version 7.0, whereas the modules will run in Windows PowerShell 5.1 or PowerShell 7.0.
I used a Windows 10 workstation for this example. Annoyingly, it came with PowerShell version 5 out of the box. Microsoft provides the latest stable version of PowerShell in a GitHub repository [2] if you need to upgrade. At the time of writing, this was the PowerShell-7.1.4-win-x64.msi
file, which let me install and start PowerShell 7.
If you want to distinguish between different PowerShell versions, you can use the $PSVersionTable
query. Note that the table created in this way returns a group of attributes with different
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
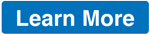