A ptrace-based tracing mechanism for syscalls
Hidden Treasures
Scientific applications can be limited by file I/O because of their distributed nature and implementation of storage. The libiotrace
library, extended with ptrace
-based syscall tracing, lets users and developers analyze file-I/O-based bottlenecks.
LD_PRELOAD
Dynamic profiling analyzes a program during runtime, thereby gathering information about resource utilization. Often, you want to know which part of a program causes the highest CPU utilization or which task uses how much memory. This information can be used either to optimize the program or to manage a running system and prevent bottlenecks. You can find a multitude of tools for gathering such data.
If the source code of the program is available, you can use a debugger or an instrumentation framework to gather resource usage information. Most compilers offer an instrumenting framework that allows you to insert profiling functionality during compile time. For example the GNU compiler collection (GCC) offers support for gprof
[1], which can be used to analyze the time spent in each part of a program.
If you don't have the source code or don't want to recompile the program in question, you can use the LD_PRELOAD
environment variable. Launching a program causes the executable to be loaded into memory and executed as a new process. All the dynamic libraries (so-called shared objects) on which the program depends are loaded into the process. Once all libraries are part of the process memory, the linker collects all exposed functions from the libraries and links them against function calls. If a function is provided by more than one library, the first match is used for linking.
The environment variable LD_PRELOAD
allows you to load and link a library before any other library is loaded, so you can use it to get
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
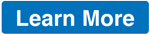