« Previous 1 2 3 4 Next »
Best practices for secure script programming
Small Wonders
Holistic system security means paying attention to even the smallest detail to avoid it becoming an attack vector. One example of these small, barely noticed, but still potentially very dangerous parts of IT that repeatedly cause serious security issues is shell scripts. Employing best practices can ensure secure script programming.
Letting Off Steam
Impressive proof of the potential harmfulness of shell scripts was provided by US software vendor Valve. The Linux-based version of the Steam game service included a script that was normally only responsible for minor setup tasks [1]. Unfortunately, it contained the following line:
rm -rf "$STEAMROOT/"*
This command, which is responsible for deleting the $STEAMROOT
directory, gets into trouble if the environment variable is not set. Bash does not throw an error but simply "disassembles" the environment variable into an empty string. The reward for this is the command
rm -rf /*.
which works its way recursively through the entire filesystem and destroys all information.
Some users escaped total ruin by running their Steam execution environment under an SELinux chroot jail. Others were not so lucky, so it is time to take a closer look at defensive programming measures for shell scripts.
Defining the Shell Variant
On Unix-style operating systems, dozens of shells are available – similar only in their support of the POSIX standard – that come with various proprietary functions. If shell-specific code from one shell is used in other shells, the result is often undefined behavior, which might not be a problem in a controlled VM environment, but deployment in a Docker or other cluster changes the situation.
The most common
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
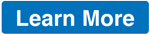