The AWS CDK for software-defined deployments
Dreaming of Clouds
The financial benefits of the cloud seem quite obvious on the surface. One powerful point that deserves not only emphasis but in-depth analysis is software-defined deployments. With the advent of cloud platforms, API-driven services and infrastructure are now the norm, not the exception, making it possible not only to automate the packaging and deployment of the software, but to provision the infrastructure that hosts the applications deployed. Although tools like Amazon Web Services (AWS) CloudFormation and HashiCorp's Terraform are heavyweights, I really think the AWS Cloud Development Kit (CDK) deserves a serious look as a strong contender in this arena.
Bring on the Clouds!
With every major cloud provider come additional benefits – in addition to the immediately obvious financial benefits – like the as-a-service offerings. For example, the Kubernetes-as-a-service offerings, such as Google Kubernetes Engine [1], Amazon Elastic Container Service for Kubernetes (EKS) [2], and Microsoft Azure Kubernetes Service (AKS) [3], make it possible to provision immensely complex orchestration platforms and tools with point-and-click web interfaces or a simple command-line invocation. In this dimension, many additional benefits that might not be immediately obvious are worth mentioning:
- Cost optimization and savings through reduction of labor. Working from the Kubernetes-as-a-service example, the time saved in not having to learn how to architect and deploy a multimaster, resilient, high-availability cluster is immense and is but one of many such services available in an easy-to-consume offering.
- Exposure and access to new technologies. It's probably fair to say that many developers would never experiment with a
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
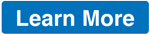