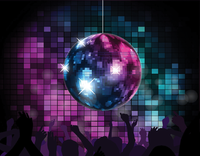
Lead Image © Laschon Maximilian, 123RF.com
Tools that extend Bash scripting
#!Bash
Admins write a great deal of code in scripting languages, and one of the most popular is Bash [1]. It has many of the basic attributes needed in a scripting language, such as variables, I/O redirection, functions, loops, conditional execution, arrays, pipes, branching, string manipulation, and so on. However, there are times when Bash can't do everything you want, and that's when you can bring in some external tools to help out.
A number of articles around the web discuss cool tricks you can do in Bash and "additions" or functions that people have created. I'll pick and choose some of these to highlight in this article. However, I must admit that I feel a little funny writing about extensions to Bash. After all, the reason Bash is so popular is that it comes with every distribution of Linux and is reasonably small and useful. Adding extensions possibly means adding new software dependencies, of which you have to be careful. With that in mind, I'll talk about some of the cooler extensions.
Pop-Up Notification Windows
One of the largest groups of extensions for Bash is GUI tools – which doesn't mean Bash can be used to code GUI interfaces, but it does allow simple GUI tools to be used within in a Bash script. This can be very useful if you are deploying an interactive Bash script for production (i.e., users).
To begin, install libnotify or libnotify-bin (for my CentOS 6 and 7 systems I installed libnotify ). Make sure your Bash script is executable, and enter:
notify-send "Dinner is served" -t 10000
You should see something like Figure 1 on the screen.
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
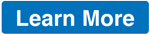