« Previous 1 2 3 4 Next »
Static code analysis finds avoidable errors
At the Source
Buffer overflow attacks have been understood since 1972, and yet buffer overflows still dominate the warning lists of security analysts today. In this article, I make a plea for compliance with coding standards, more and better source code reviews, and the use of good tools for static analysis that can improve quality and security.
Lack of training and inadequate quality assurance and checks are the root causes of breakdown-prone software. As early as 1972, scientists described the first, albeit theoretical, buffer overflow attack [1], and SQL injections [2] have been around since 1998. Both still account for the majority of IT security vulnerabilities today, and both can be easily avoided: buffer overflows with n
functions (e.g., strncpy()
instead of strcpy()
) and SQL injections with prepared
statements. But who makes sure developers adhere to these practices?
Although coding standards that prescribe secure functions would help avoid repeating these classic errors, hardly any company or project even lays down mandatory rules, let alone monitors compliance with them. Therefore, very few developers tend to orient their work on security targets.
Brackets and Indentation
Having to check manually whether a fellow programmer has, for example, correctly indented their curly braces is unlikely to make code review a more attractive task. Tools can and should do this kind of no-brainer work, but they have to be flexible.
Opinions differ, even in terms of the visual arrangement of curly braces and their indentation depth. Although I can never completely shake off my Pascal past and always start a block in a new line with a bracket (Pascal begin
style), many view the loop header or the condition as sufficient and
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
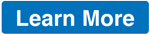