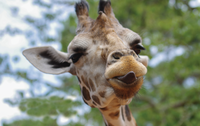
Photo by Aidas Ciziunas, Unsplash.com
Haskell framework for the web
Speaking in Tongues
Frameworks for the web are a dime a dozen. Most are based on the PHP and JavaScript programming languages, whereas others use Python, Ruby, Go, or other such languages. When a developer uses WordPress [1], Drupal [2], or Joomla [3], generally they only have to unpack the core on the server and then add other modules. Installing security updates conscientiously and in good time counteracts ubiquitous malware threats.
Quite a few reasons speak to the use of exotic frameworks such as the Haskell-based Yesod [4] [5]. It is not just that Yesod is less widespread, it is also that the security built into the compiler (Haskell is type safe) makes attacks time-consuming or impossible, and SQL and JavaScript injections unlikely. Additionally, the Glasgow Haskell Compiler (GHC) [6], version 1.0 of which has existed since April 1990, is based on the fast C compiler and processes requests to the website far more efficiently than many other programming languages. Because Haskell is a functional programming language, it facilitates other tasks, such as multithreading.
Developers mainly ask themselves one question: How do I get started with the framework? Haskell is considered difficult to learn, because it is strongly orientated on mathematical logic and uses many abstract concepts like monads and category theory. A framework like Yesod helps developers make the transition to Haskell and levels a still steep learning curve, thanks to pre-built components that promise quick results. In this article, I will help you take your first steps with Yesod.
Installation
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
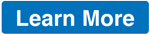