« Previous 1 2
The Lua scripting language
Moonstruck
The Lua scripting language celebrates its 20th birthday this year. Because it is used more often as an embedded scripting language than as an independent programming language (e.g., Python or Perl), Lua is less familiar to many people.
Lua [1] is very widespread in games and game engines. In fact, Wikipedia lists nearly 150 games that use Lua in a separate category of "Lua-scripted video games." However, Lua can also be found in a variety of network and system programs, such as the Wireshark network analyzer, the Nmap scanner [2], the MySQL Proxy program, the Rspamd anti-spam solution, the FreeSWITCH VoIP software, the Redis NoSQL database, the Apache web server [3], and the Nginx reverse proxy service (see the OpenResty article in this issue).
Homegrown
Lua was developed at the Catholic University of Rio de Janeiro by Roberto Ierusalimschy, Luiz Henrique de Figueiredo, and Waldemar Celes. Because Brazil was subject to strong import restrictions for hardware and software in 1992, the three decided to develop a separate scripting language for their own purposes, which eventually culminated in Lua (Portuguese for "moon"). Ierusalimschy still controls the development today and has published the standard work Programming in Lua , third edition, which was published early last year. The first edition, available online [4] refers to Lua 5.0. The language has now moved to version 5.2, but the online book is still up to date to a large extent.
As mentioned, Lua is primarily designed as a library that application programmers can integrate into their software to add scripting capabilities. However, you can also use Lua without additional software. The
...Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
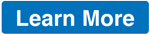