« Previous 1 2
Isolate workloads from Docker and Kubernetes with Kata Containers
Sealed Off
If DevOps adherents want to package and run applications separately, as the paradigm of continuous delivery dictates, they encounter a problem. Either they host their workloads on physical or virtualized servers in the old-fashioned way, or they entrust data and programs to the kind of containers that have recently become popular under the Docker label. Both approaches have advantages and disadvantages.
The advantage of physical servers is the high degree of isolation. In the case of a bare metal device, this is immediately understandable; for a virtual machine (VM), the hypervisor handles separation with the help of its processor. It's a peculiar twist of fate that the Meltdown and Spectre vulnerabilities have shown that even hardware is not infallible, but disregarding this unpleasant chapter on the limits of engineering skill, both servers and VMs effectively separate resources by sharing only the CPU and no other resources.
In contrast, all containers running on one host use the same kernel. When the individual workloads are small and plentiful, the savings potential is considerable, particularly with modern cloud-native applications that run as microservices.
Isolation of Resources
Data isolation is handled by a relatively new mechanism in the Linux kernel. Namespaces keep a record within the kernel of which process is allowed to access which resources (e.g., processes, network interfaces, or hard disk directories). Docker instrumentalizes these namespaces and controls them and a few other mechanisms with a convenient command-line tool.
DevOps thus effectively has the choice between heavyweight VMs that take a long time to boot and lightweight containers with limited isolation that rely on cleverly implemented kernel-based isolation.
Starting from this situation, several initiatives have tried to combine the two approaches. As a by-product of its Hyper-V
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
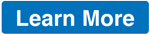