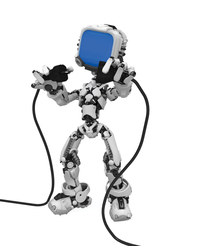
Lead Image © higyou, 123RF.com
Internet socket programming in Perl
Plugged In
Some would make the case that Perl is the most used and most capable scripting language on the Internet today, and this is probably true. Its versatility and ease of use has made it an industry standard used throughout the world.
With Perl sockets, you can communicate with any computer that is connected by the Internet or a network. To communicate with this other computer, you specify the server to connect to and a port to connect through. After the initial connection, you can use that connection just as you would a file handle, with just a few differences that are mainly academic.
To put it simply, the two sides of a Perl socket are the server and the client. It doesn't really matter which is which – the communication process is essentially the same, because the client can send information to the server and vice versa. The communication process is surprisingly simple. The process starts with the creation of the server. The server patiently waits for a connection request from the client, which can be created any time after the creation of the server. The client connects to the server with the address of the server and the mutually agreed upon port number. If the port numbers are different, no connection is made. Once the client is connected, data may be sent from the server to the client, and vice versa.
Data can be sent over a socket two ways: both ways using one socket, or one way using two sockets. In the one-socket method, data is sent from the server to the client and from the client to the server through the same socket. In the two-socket method, one socket is used to send data from the server to the client, and one is used to send data from the client to the server. I'll be touching on both methods in this article, so read on.
Sockets exist on all major operating systems (OSs). They are the accepted way to achieve communication between computers. Keeping this in mind, you can connect a Unix computer to a Windows computer (and vice
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
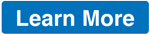