Advanced MySQL security tips (a complete guide)
Guard Duty
MySQL security configurations include a range of topics, along with their possible effects on MySQL servers and corresponding applications. In this article, I look at MySQL security encryption services, account-associated authorization systems, and other required security precautions to ensure protection against misuse and attacks. This security guide will help you protect sensitive data, even if the MySQL service is compromised at some point.
Most of the advanced MySQL security configurations require changes to the server's main configuration file my.cnf
. This file is generally located inside the /etc/mysql
directory or in the /opt/lampp/etc/
folder for LAMPP installations. However, you can locate the file with the find
or locate
command in Linux.
Encryption at Rest
By default, MySQL stores plaintext data inside InnoDB tables. Any non-admin user with access to these files can read tables and pose a security threat. Data at rest or in transit both require protection to avoid potential risk. MySQL transparent data encryption (TDE) enables encryption at rest to prevent information threats and privacy breaches, even if your system is compromised at some level.
MySQL provides TDE by enabling encryption at rest for physical files in the database. It encrypts data automatically on the go before writing to the storage and decrypts before reading. In this section, I will show you how to configure encryption at rest to ensure protection from physical data theft.
Getting Started
MySQL InnoDB tablespaces are stored in .ibd
file format and are generally located in the /var/lib/mysql
directory. You can also find them with the locate
command:
$ locate ibd |...
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
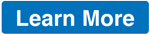