« Previous 1 2 3 Next »
Policy rulesets in cloud-native environments
Just Enough
Infrastructure as Code (IaC) has become a successful recipe for declarative, machine-readable code, so it only makes sense to apply this system to security and, in particular, to authoring policies in an attempt to implement rules within an organization in a scalable way. One representative of this genre that has recently received greater attention is the Open Policy Agent (OPA) project [1], which is backed by startup Styra. OPA is a general-purpose policy engine that enables consistent, context-aware policy enforcement across the stack.
OPA at a Glance
OPA is hosted by Cloud Native Computing Foundation (CNCF), the organization behind Kubernetes. Designed for cloud-native environments, OPA combines the relatively easy-to-learn and -read Rego policy language with a policy model and application programming interface (API), which allows for a kind of universal framework that applies rules to any kind of stacks. One of the great advantages of OPA is the ability to decouple security policies from code and its use – regardless of how often the code changes.
From a technical point of view, OPA is tied to the input. Once data is available, the OPA code decides how to handle it (e.g., allowing or blocking with an allow or deny policy). Another advantage is that OPA processes take input and create output in both JSON and YAML formats, meaning that IT managers do not have to stick to a predefined API. All told, writing rules is relatively easy, and OPA supports read, evaluate, print, and loop (REPL, i.e., shell-based code execution). Of practical value is that you do not have to write all the policies yourself, because you can easily find ready-made policy bundles online for many use cases, and they are likely to contain a useful, predefined set of rules. A freely accessible Playground [2]
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
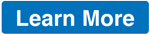