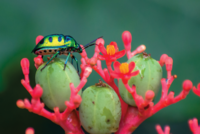
Photo by Sonika Agarwal on Unsplash
A Distributed SQL Database
Insect Collector
CockroachDB [1] is an open source, distributed SQL database that provides scalability, high availability, and strong data consistency across multiple data centers and cloud environments. It was designed by former Google employees who later founded the company Cockroach Labs. CockroachDB is built on the foundation of the Google Spanner research and is often referred to as "Spanner for the masses" (see the "What is Google Spanner?" box), making the power of distributed databases accessible to a broader audience.
What is Google Spanner?
Google Spanner is a database management system that stands out from other database systems in several ways. It is designed to handle large amounts of data and high transaction rates at a global scale, with automatic sharding, distribution, and replication of data across multiple regions.
Spanner provides strong consistency guarantees, ensuring that all nodes in the system have the same view of the data at the same time, making it ideal for mission-critical applications. It also uses an SQL-based query language, making it easy for developers to interact with the database with familiar tools and APIs. Additionally, it supports ACID (atomicity, consistency, isolation, durability) transactions, which maintain data integrity in the face of system failures or concurrent transactions.
Spanner supports multiregion replication, which allows data to be replicated across multiple regions for low-latency access to data and increased reliability. Furthermore, it supports horizontal scaling, enabling it to add more nodes to the system to handle increasing workloads and scale up or down according to the application's needs, making it a powerful and unique database management system.
Unfortunately, it was never open sourced – it is a proprietary database system developed and operated by Google. The only way to access it is to subscribe to Google Cloud Platform. However, Google has published several papers and technical documents that describe the system's design and architecture, which proved helpful for researchers and developers interested in distributed systems and database management and inspiring solutions such as CockroachDB.
One of the key features of CockroachDB is its horizontal scalability. As your application grows, you can add more nodes to the cluster easily without any downtime, allowing the database to rebalance and distribute the data automatically across the new nodes. In this way, CockroachDB can handle increasing workloads efficiently without compromising performance.
CockroachDB uses a strong consistency model to ensure data integrity and provides ACID-compliant transactions, which are essential for maintaining data consistency in applications that require complex operations. The database achieves this state by using a consensus algorithm called Raft to keep replicas synchronized and ensure that all updates are atomic, consistent, isolated, and durable.
To give you a sense of how you can interact with CockroachDB, I'll look at some basic code examples. After installing the software (see the Deployment section below), start a local single-node CockroachDB cluster with the command:
cockroach start-single-node --insecure --listen-addr=localhost
Next, you can interact with the database from the built-in SQL shell, just as with MySQL and PostgreSQL:
cockroach sql --insecure --host=localhost
Note the --insecure
option, which is used for simplicity. In production environments, you should use a secure connection that involves more elaborate preparations (e.g., generating certificates). A detailed guide for the most recent versions of CockroachDB can be found on the project website [2].
The next step is to create a simple database schema and insert some data. In this example, you'll create a database for a bookstore, with a table for books (Listing 1), and insert a few records into the books
table (Listing 2).
Listing 1
Sample Table
CREATE DATABASE bookstore; USE bookstore; CREATE TABLE books ( id UUID PRIMARY KEY DEFAULT gen_random_uuid(), title STRING NOT NULL, author STRING NOT NULL, publication_date DATE, price DECIMAL(10, 2) );
Listing 2
Inserting Sample Books
INSERT INTO books (title, author, publication_date, price) VALUES ('The Catcher in the Rye', 'J.D. Salinger', '1951-07-16', 15.99), ('To Kill a Mockingbird', 'Harper Lee', '1960-07-11', 12.99), ('Pride and Prejudice', 'Jane Austen', '1813-01-28', 9.99);
At this point you can run a simple query to retrieve the data:
SELECT * FROM books;
As you can see, CockroachDB can be used just like a traditional SQL database, with little difference in basic functionality from other, non-distributed solutions. Accessing CockroachDB from programming languages is equally simple. For example, in Python, you can use a popular database library such as psycopg in much the same way you would deal with MySQL or PostgreSQL, despite your data being in data centers in various parts of the world.
Distributed Systems
Before I move on, you need to understand what is meant by distributed systems in a more formal framework. Generally speaking, distributed systems consist of multiple interconnected computers or nodes that work together to achieve a common goal, such as processing and managing large amounts of data. In the context of databases, distributed systems enable the storage and management of data across multiple nodes, providing better performance, scalability, and fault tolerance compared with traditional monolithic databases – at least in theory.
Distributed systems have several advantages in database management, if done well. First is scalability: Opposed to traditional SQL DBMSs, distributed systems can easily scale horizontally by adding more nodes to the cluster, which allows them to handle increasing workloads and data sizes without degrading performance. In contrast, scaling a monolithic database often requires upgrading hardware, which can be expensive and disruptive. The other approach is to partition the database (e.g., by sharding, often resulting in unbalanced tables).
Another advantage is high availability: In a distributed system, data is typically replicated across multiple nodes, ensuring that the system remains operational even if some nodes fail. This redundancy provides fault tolerance and helps maintain service continuity in the face of hardware failures, network issues, or other disruptions. Moreover, distributed systems can distribute workloads across multiple nodes, allowing them to balance the load and provide better performance. This can be particularly beneficial for read-heavy or write-heavy applications, where distributing the workload can help avoid performance bottlenecks.
As an additional advantage, in geographically distributed systems, data can be stored closer to the users or applications that need it, reducing latency and improving performance. This can be especially important for global applications, where users might be located in different regions around the world.
The CAP Theorem and CockroachDB
The CAP theorem, also known as Brewer's theorem, is a fundamental principle in the field of distributed systems. It states that it is impossible for a distributed data store to provide consistency, availability, and partition tolerance (CAP) simultaneously and that a system can only guarantee two out of these three properties at any given time. In the context of the CAP theorem, the terms are defined as follows:
Consistency: Every read operation in the system will see the most recent write or an error. In other words, all nodes in the system agree on the current state of the data.
Availability: Every non-failing node in the system will respond to read and write requests in a reasonable amount of time, which means that the system is always up and running and can process user requests.
Partition tolerance: The system can continue to function despite network partitions, meaning that the system can tolerate a failure of network communication between nodes in the system.
CockroachDB is designed to prioritize consistency and partition tolerance (CP) while still providing a high level of availability (A). It achieves this state by using the Raft consensus algorithm to manage data replication and ensure strong consistency across nodes. In the event of network partitions, CockroachDB favors consistency over availability, ensuring that data remains consistent even if some nodes become temporarily unreachable.
To demonstrate how CockroachDB handles network partitions and maintains consistency, I'll walk through a simple code example. Assume you have a three-node CockroachDB cluster, and the books
table created earlier (Listing 1).
First, check the replication factor for your table. By default, CockroachDB replicates each range of data three times. You can verify this by running the query
SHOW EXPERIMENTAL_REPLICA CONFIG FOR TABLE books;
Now, simulate a network partition by stopping one of the nodes in your cluster. This action will leave you with only two nodes out of three being operational. You can stop a node by issuing the command
cockroach quit --insecure --host=<node_address>
Despite the network partition, CockroachDB continues to operate, ensuring that your data remains consistent. You can still perform read and write operations on the remaining nodes:
INSERT INTO books (title, author, publication_date, price) VALUES ('1984', 'George Orwell', '1949-06-08', 14.99); SELECT * FROM books;
As long as a majority of the replicas (in this case, at least two out of three) are operational, CockroachDB can continue processing transactions, providing a high level of availability.
Once the network partition is resolved and the stopped node comes back online, CockroachDB automatically synchronizes the data across all nodes:
cockroach start --insecure --join=<node_address_1>,<node_address_2>,<node_address_3>
This ensures that the previously stopped node receives any updates that occurred during the partition.
Architecture
The CockroachDB architecture is designed to be highly scalable, fault-tolerant, and consistent. At a high level, it comprises several core components that work together to manage the storage, distribution, and processing of data.
CockroachDB organizes its data into a single, monolithic, ordered key-value store called the KV layer. This key-value store is then divided into smaller, contiguous chunks called ranges, which are typically 64MB in size. These ranges are replicated across multiple nodes by the Raft consensus algorithm, ensuring strong consistency and fault tolerance.
On top of the KV layer, CockroachDB implements an SQL layer that provides support for SQL operations and transactions. This layer translates SQL queries into key-value operations that can be executed on the underlying KV store.
To explore how CockroachDB processes a very simple SQL query, like the one used earlier to retrieve all the books in the books
table, use
SELECT * FROM books;
When a client submits this query, it is first parsed and optimized by the SQL layer. The optimized query is then translated into a series of key-value operations that can be executed on the KV store.
The query execution engine in CockroachDB is responsible for coordinating the execution of these key-value operations across the nodes that store the relevant ranges. To do this, it first locates the nodes holding the replicas of the data being queried by consulting the cluster's meta ranges, which store information about the location of all the ranges in the system.
Once the appropriate nodes have been identified, the query execution engine sends the key-value operations to these nodes for execution. The nodes then return the results to the query execution engine, which aggregates and processes the data before returning the final result to the client.
During this process, CockroachDB ensures that all operations adhere to its strong consistency and transactional guarantees. For example, if a transaction involves multiple key-value operations, CockroachDB uses a combination of the Raft consensus algorithm and a distributed transaction protocol called the two-phase commit (2PC) protocol to ensure that these operations are executed atomically and consistently across all the involved nodes.
Here's a simple example of a transaction that involves updating the prices of two books:
BEGIN; UPDATE books SET price = price * 0.9 WHERE title = 'The Catcher in the Rye'; UPDATE books SET price = price * 0.8 WHERE title = 'To Kill a Mockingbird'; COMMIT;
CockroachDB ensures that either both price updates are applied or neither of them are, maintaining the consistency and integrity of the data.
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
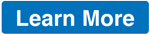