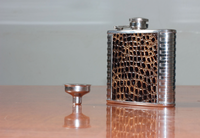
© Photographer, 133RF.com
Web applications with Flask
A Tasty Drop
If you are considering writing a web application with Python, one obvious choice is Django, which enjoys popularity within the Python world that is similar to Rails in the Ruby community. An alternative to Django is Flask [1], which has become increasingly popular since its inception in 2010.
The advantage of Django is that it comes with more or less everything you need for many web applications: templates, a database connection, user authentication, and so on. However, it is difficult to leave out or replace components. If you prefer a more flexible approach, you should take a look at Flask. Out of the box, Flask relies on underpinnings provided by a WSGI library by the name of Werkzeug [2], which was written by Flask programmer Armin Ronacher. The same applies to the Jinja2 template library, which Flask uses by default.
WSGI [3] is a specification that defines how a web server software and a web application written in Python communicate. This could be the Apache or Nginx web server with their WSGI modules or special WSGI web servers, such as Gunicorn or uWSGI. The Flask library also contains a separate web server for development.
The latest version of Flask, 0.9, was released in July 2012, and it is uncertain when version 0.10 will be released. Flask requires at least Python 2.5; Python 3 is not currently supported. The easiest approach is to install Flask with Virtualenv [4], which maintains a local version of Python and its modules in a local directory, thus avoiding conflicts with the other projects on a server. Virtualenv itself is available with most Linux distributions. Alternatively, you can install it with the Python package management tools pip
and easy_install
.
The following commands create
...Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
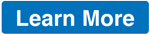