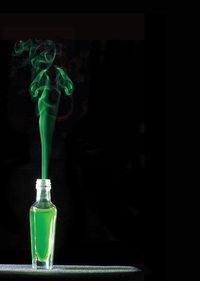
© Egor Arkhipov, 123RF.com
A web application with MongoDB and Bottle
Genie in a Bottle
The usual suspects for open source database systems were easily identified in the past, but these days, things have changed. Instead of MySQL or PostgreSQL, people are talking about CouchDB, FluidDB, and MongoDB. These databases have a feature scope similar to classical SQL servers but offer far better performance and scalability. Although they can't compare with systems simple key/value store systems such as Memcached, this disadvantage is easily offset by the better feature scope in combination with what is still excellent performance. On top of this, the performance of these systems scales well horizontally. Instead of needing to buy expensive hardware, you can easily distribute a database over multiple systems without affecting functionality.
MongoDB is already on board with any major Linux distribution. In combination with Python and a web framework, you can thus quickly achieve impressive results. In this example, I will be using the Bottle micro web framework [1]. This very simple framework comprises a single file, which you can drop onto your own system using easy_install
.
The Bottle framework is perfect for initial tests with MongoDB because there are no dependencies to resolve, except for the Python standard library, and you still get a fair amount of functionality for your money. It supports templates and comes with its own web server. The interface between the MongoDB service and the Bottle web framework in this example is PyMongo [2], which is also available out of the box with most distributions.
MongoDB splits a database into collections, which in turn store documents. The documents contain the database objects in JSON format. The good point is that the collections are not governed by a schema. Thus, you can simply transfer the desired data to the database without worrying about modifying the schema, which makes
...Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
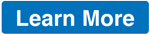