« Previous 1 2 3 4 Next »
ASP.NET web development framework
Common Core
Small and large web applications are becoming increasingly common in the enterprise. Many administrators program small tools to optimize existing system management, and Microsoft's .NET Core now makes this possible across operating system boundaries. In this article, I look at the part of the .NET Core functionality that enables the development and operation of dynamic web applications – ASP.NET Core – and its use on Linux, with a focus on the peculiarities of providing ASP.Net Core applications and not on their application logic or graphical design.
.NET Core Genesis
At the beginning of the century, Microsoft heralded a new era in their software development history with the announcement of the .NET Framework. A promise was made that the framework would not be exclusively reserved for the Windows platform. After all, the write once, run anywhere (WORA) principle was one of the factors that had already helped Java achieve great success as an enterprise platform.
Microsoft did not pursue the "Linux.NET" idea any further although several open source projects emerged to enable seamless porting of .NET applications to Linux and Unix. The best known and longest lasting of these projects was Mono, developed and maintained by the core team that later created Xamarin; however, Mono never achieved true feature parity with the official .NET.
The deep divide with Linux and open source seems to have largely been overcome at Microsoft. Xamarin is now also based in Redmond and the company's products have been incorporated into Visual Studio as components for the development of mobile applications. In view of this openness toward other platforms, the idea of extending the leading development framework to Linux, macOS, and potentially CPU architectures other than x64 and x86 was obvious.
Microsoft decided to redevelop the framework completely, with an open source and
...Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
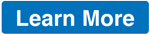