« Previous 1 2
Retrieving Windows performance data in PowerShell
Taking Precautions
Basic performance data and health status of Windows computers can be collected and evaluated very easily and conveniently using PowerShell. The scripting language is an ideal candidate both for local and remote machines because it is familiar with a variety of interfaces in different Windows subsystems.
The number of interfaces and connection points increases steadily with each new version of a Microsoft product. For example, Windows performance, process, registry, and network information is easily understood using Windows Management Instrumentation (WMI). Data from Windows server roles and from the operating system's advanced configuration do not pose any obstacles, either.
In this article, I show you how to use PowerShell to access WMI and performance data on local or remote computers, how to find relevant WMI objects, and how to look at the performance of individual computers and check for violations of threshold values. The scripts shown here are intended for Windows installations starting from Windows 7 and Windows Server 2008 R2.
Collecting WMI Object Data
Much of the information available via a computer can be accessed or controlled as objects automatically via the WMI interface. The interface publishes information about the computer, the operating system, and objects, which can be queried in the form of classes and attributes. WMI also can control your computer remotely. Some classes implement methods that can be used to shut down the computer or configure operating system components.
WMI is a good candidate for a general inventory of machine properties because of the extensive amount of data provided. Of course, PowerShell provides the necessary tool to collect the data. To access WMI objects, you use the Get-WMIObject
cmdlet with the WMI object to be queried as a parameter (Listing 1).
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
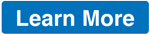