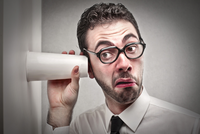
Lead Image © bowie15, 123RF.com
Features of PowerShell loops
Say Again?
Microsoft has emphasized that the increased use of the PowerShell scripting language will make life easier for system managers. After all, maintaining and operating Windows systems means above all that many tasks need to be handled regularly and for a large number of computers. Just as robots tirelessly fit doors and windshields on assembly lines, small and not-so-small scripts can handle recurring work, such as creating users, monitoring processes, or installing software.
Any programming or scripting language needs instructions the user can employ to design loops. It is irrelevant whether the language comes from the structured days of old or is one of the latest generation of object-oriented languages. PowerShell would not be PowerShell if it did not offer several approaches and options for almost every task and requirement – including loop constructs. Beginners thus first need to struggle with the distinction between the for
loop, the foreach
construct, and the foreach-object
cmdlet – not forgetting the do
and while
loops, which also can be interrupted as needed.
The for Loop
Anyone who has ever programmed a computer is probably familiar with for
loops. Using this construct lets arbitrary commands reiterate within a script block, as long as a previously defined condition is true. The basic structure looks like this:
for(<start value>; <run condition>; <iteration>) {commands}
Use of this kind of loop is especially useful when the programmer knows exactly how often the loop should iterate. The following example creates exactly four text files with a corresponding index:
for($count=1; $count -lt 5; $count++) {New-Item -Path h:\tmp -name "file$count.txt" -itemtype file}
The variable $count
is initially
Buy this article as PDF
(incl. VAT)
Buy ADMIN Magazine
Subscribe to our ADMIN Newsletters
Subscribe to our Linux Newsletters
Find Linux and Open Source Jobs
Most Popular
Support Our Work
ADMIN content is made possible with support from readers like you. Please consider contributing when you've found an article to be beneficial.
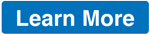